Sentiment Analysis using Azure Text Analytics
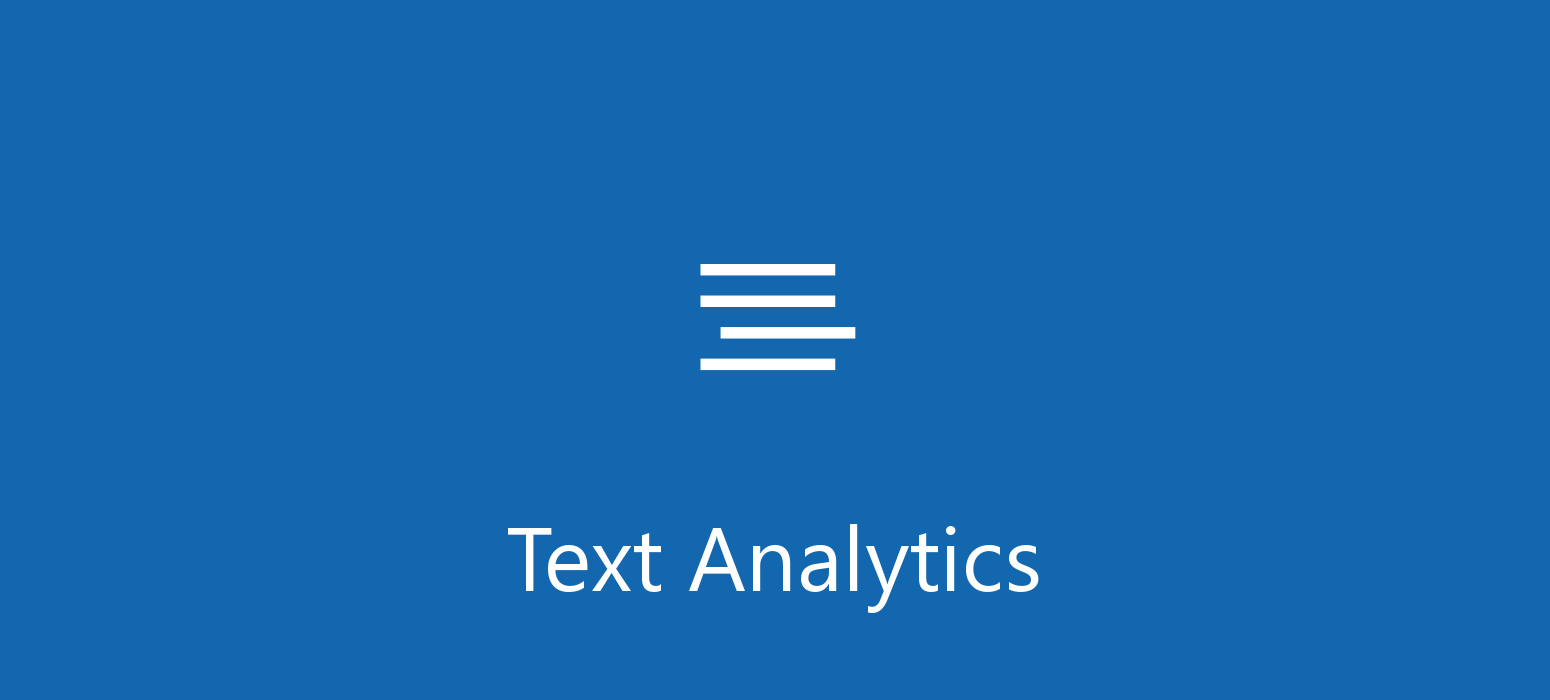
Text Analytics is an easy to learn and fast to implement AI service, part of the Azure Cognitive Services, that uncovers insights such as sentiment, entities, relations and key phrases in unstructured text.
Create
To create a text analytics resource go to Azure Portal and search for Text Analytics. Select Text Analytics from the Marketplace.
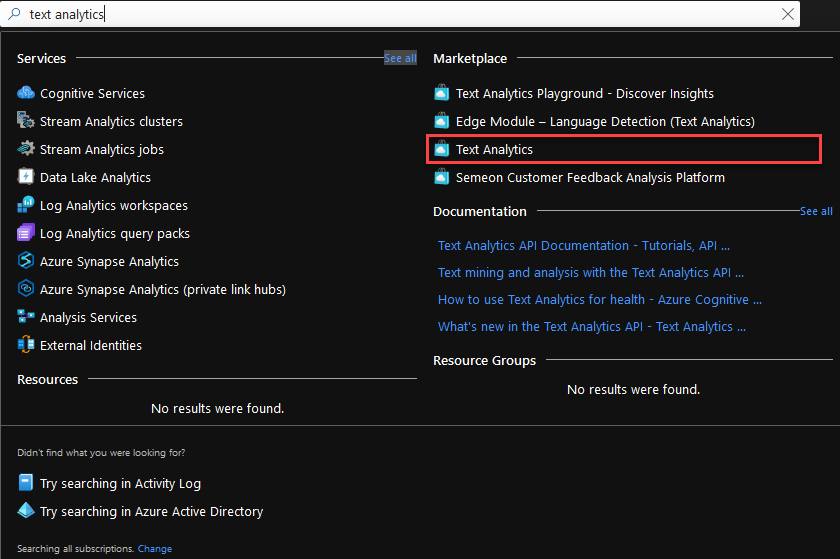
Fill the form. The fields with the ‘*’ are mandatory.
- Subscription should already be filled in with your default subscription.
- For Resource Group, you can use an existing one, or create a new one.
- You can leave Region with the pre-selected region.
- Name is the name of your new Text Analytics resource.
- Any Pricing tier will do for this demo.
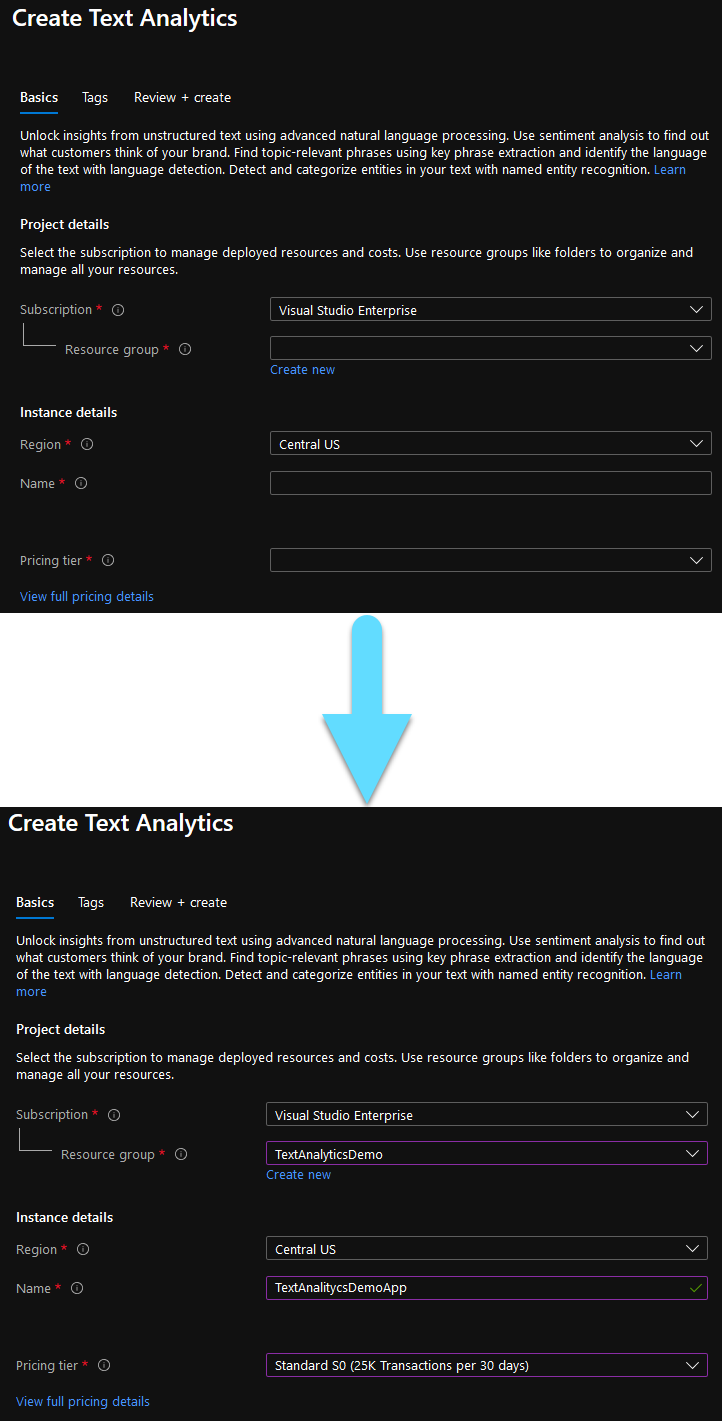
Continue to the last step and click Create.
Go to your resource and navigate to the Keys and Endpoint tab at the left of your window. From here you can grab a Key and your Endpoint. You will need theese later.
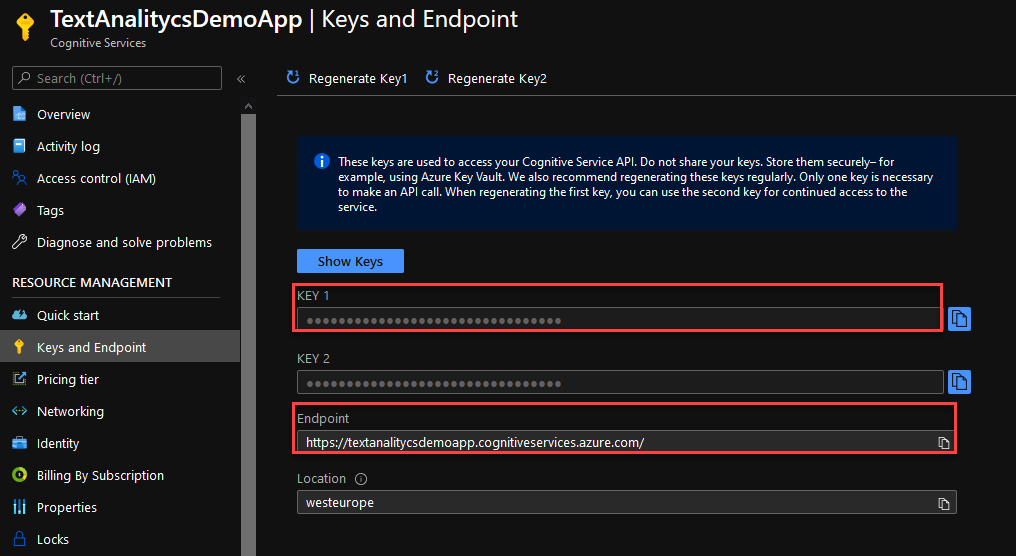
Implement
Open your existing project in Visual Studio. If you are not working on an existing project, simply create a C# (.NET Core) Console app.
Navigate to Project -> Manage NuGet Packages, find and install the Azure.AI.TextAnalytics package.
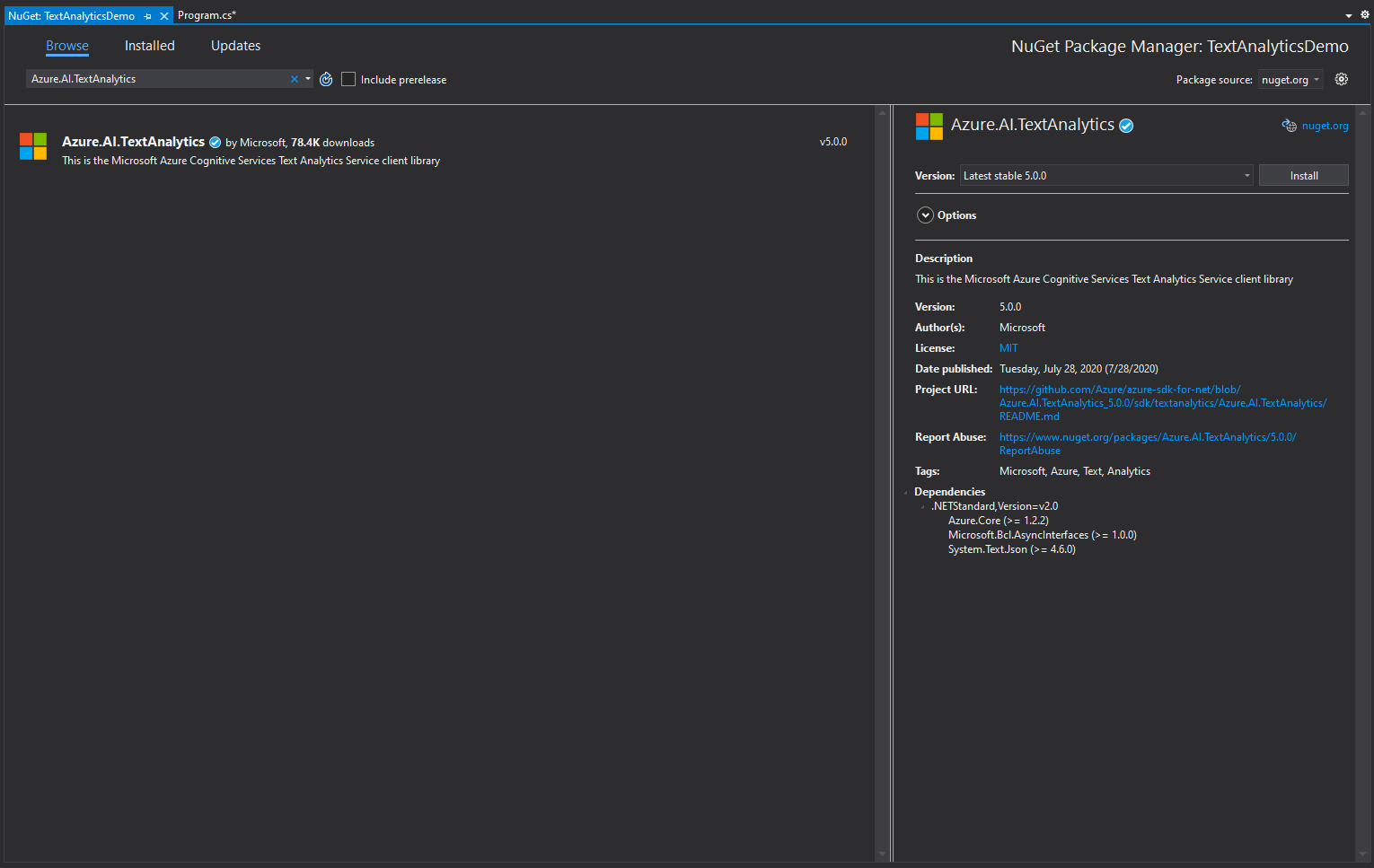
Open the class that you need to implement the sentiment analyser in. For a new project you can use the Program.cs.
Add the using statements you see below at the top of the file.
using Azure; using Azure.AI.TextAnalytics;
Add your credentials and endpoint at the top of your class. For the string in credentials you can use one of the keys you got before and the endpoint for the string in endpoint.
private static readonly AzureKeyCredential credentials = new AzureKeyCredential("30da76KEY_HERE5ef63b3993f03"); private static readonly Uri endpoint = new Uri("https://textanalitycsdemoapp.cognitiveservices.azure.com/");
Inside your Main put the code that appears below. Do not worry about the missing function, we will create it in the next step.
static void Main(string[] args) { var client = new TextAnalyticsClient(endpoint, credentials); SentimentAnalysisExample(client); }
The SentimentAnalysisExample is the function that analyses the text and determins weather the intention was positive or negative. In the inputText string at line 3 you can put the document you want to analyse. Every sentense will be analysed separately and a overall result for the whole document will appear.
static void SentimentAnalysisExample(TextAnalyticsClient client) { string inputText = "I am feeling happy. I am sick."; DocumentSentiment documentSentiment = client.AnalyzeSentiment(inputText); Console.WriteLine($"Document sentiment: {documentSentiment.Sentiment}\n"); foreach (var sentence in documentSentiment.Sentences) { Console.WriteLine($"\tText: \"{sentence.Text}\""); Console.WriteLine($"\tSentence sentiment: {sentence.Sentiment}"); Console.WriteLine($"\tPositive score: {sentence.ConfidenceScores.Positive:0.00}"); Console.WriteLine($"\tNegative score: {sentence.ConfidenceScores.Negative:0.00}"); Console.WriteLine($"\tNeutral score: {sentence.ConfidenceScores.Neutral:0.00}\n"); } }
If you wish to add extra functionality to your text analyser you can add functions from here and call them from Main. You can try Opinion mining, Language detection, Named Entity Recognition and many more.
Test
Now simply run the program to see how well the sentiment analysis works. You can try changing the text to test it. Here is the output of the text we had above.
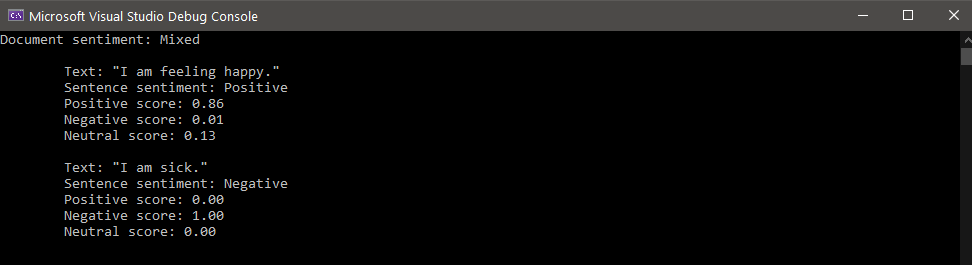
And thats how you implement sentiment analysis into your project in a few easy steps!