Give voice to your project using Azure Speech
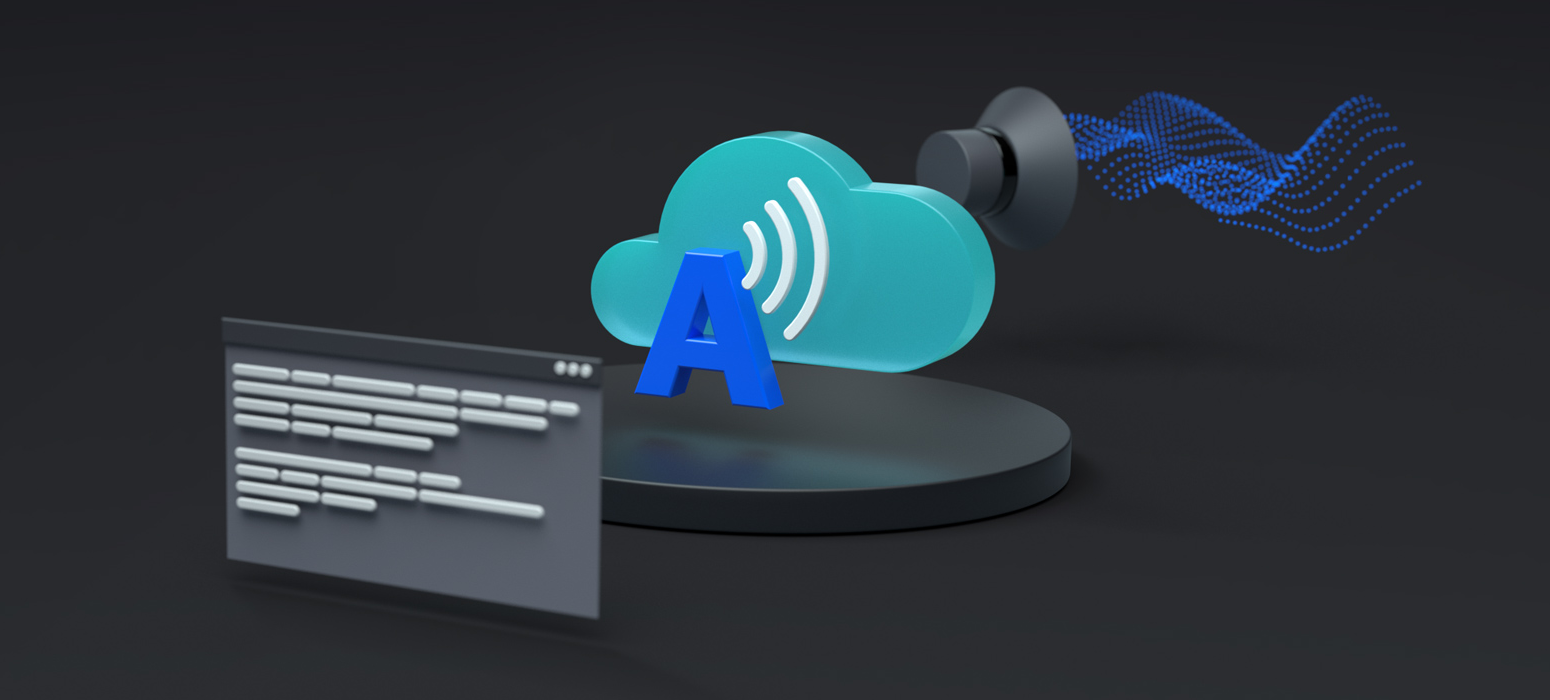
Speech is an Azure service part of the Cognitive Services that converts text to lifelike speech.
Create
Go to Azure Portal and search for Speech. Select Speech from the Marketplace.
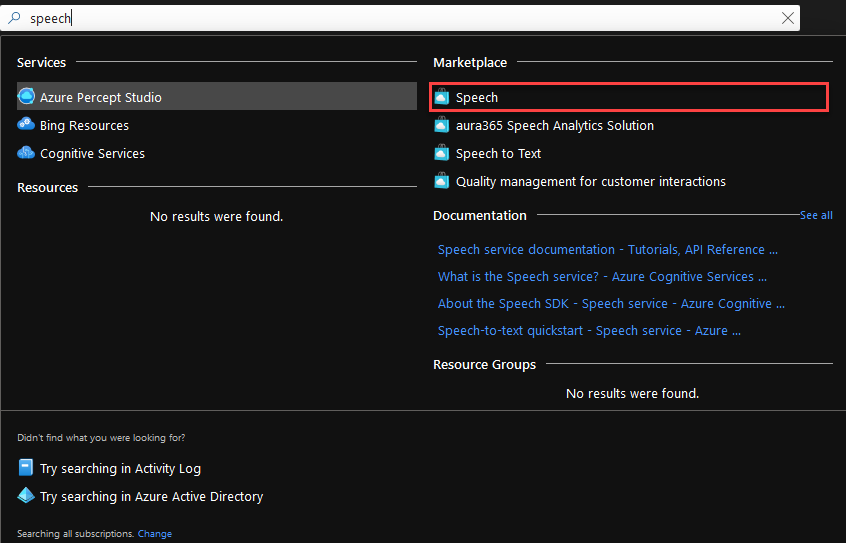
Fill the form. The fields with the ‘*’ are mandatory.
- Name is the name of your new Speech resource.
- Subscription should already be filled in with your default subscription.
- You can leave Region with the pre-selected region.
- Any Pricing tier will do for this demo.
- For Resource Group, you can use an existing one, or create a new one.
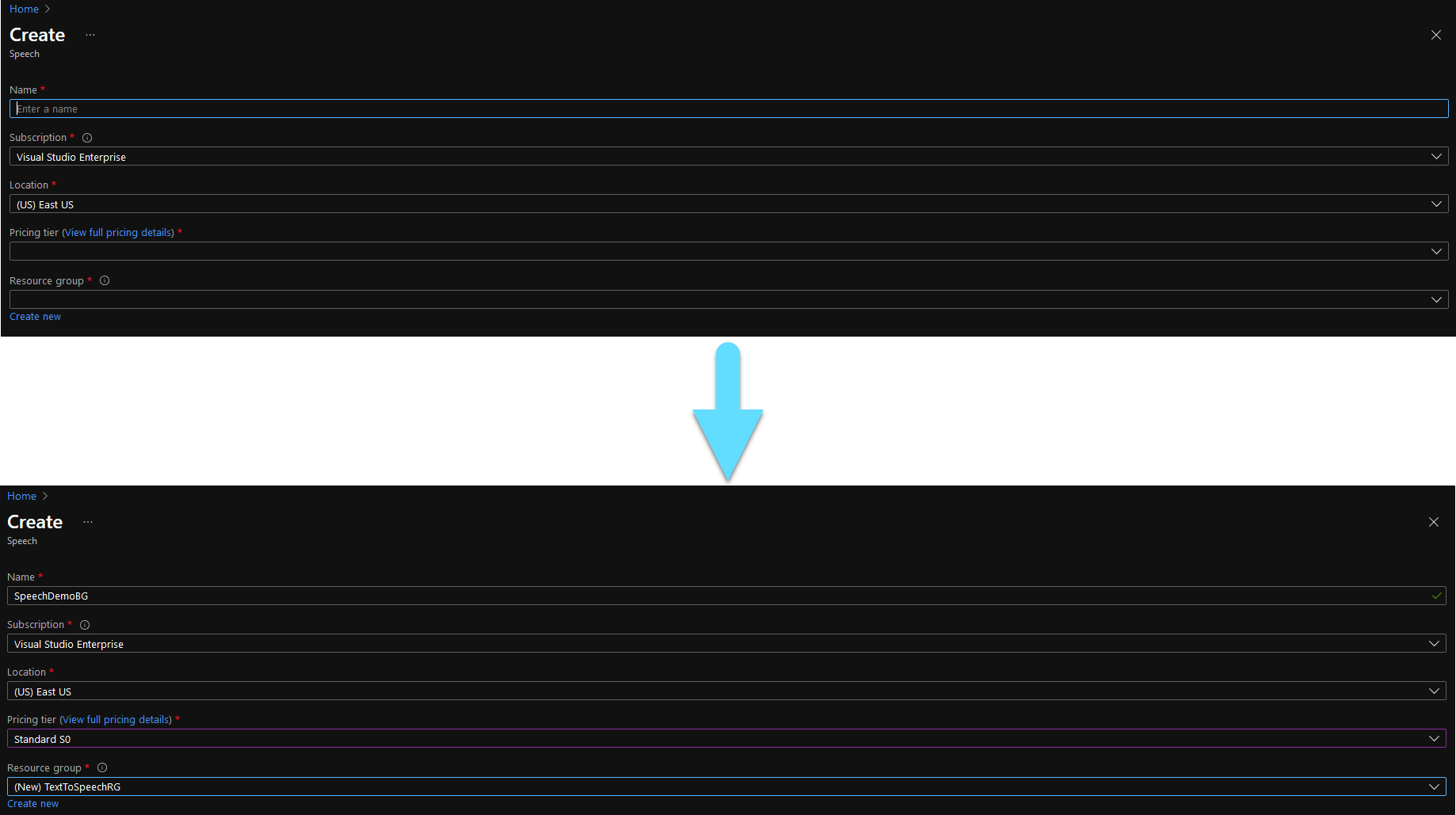
Click Create to deploy your resource. This might take a few minutes. After the deployment is done click Go to resource.
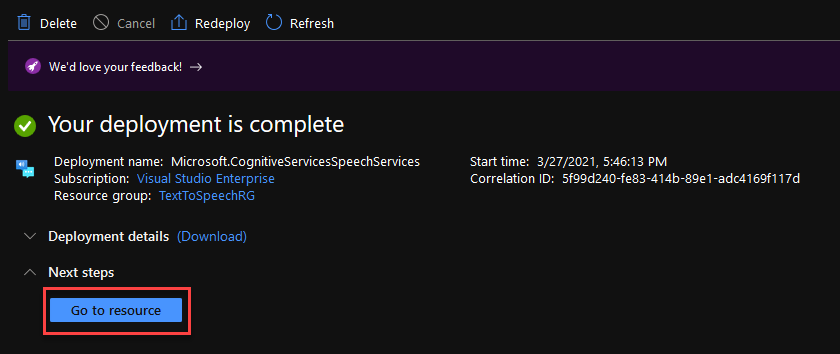
Navigate to the Keys and Endpoint tab at the left of your window. From here you can grab a Key and your Location. You will need theese later.
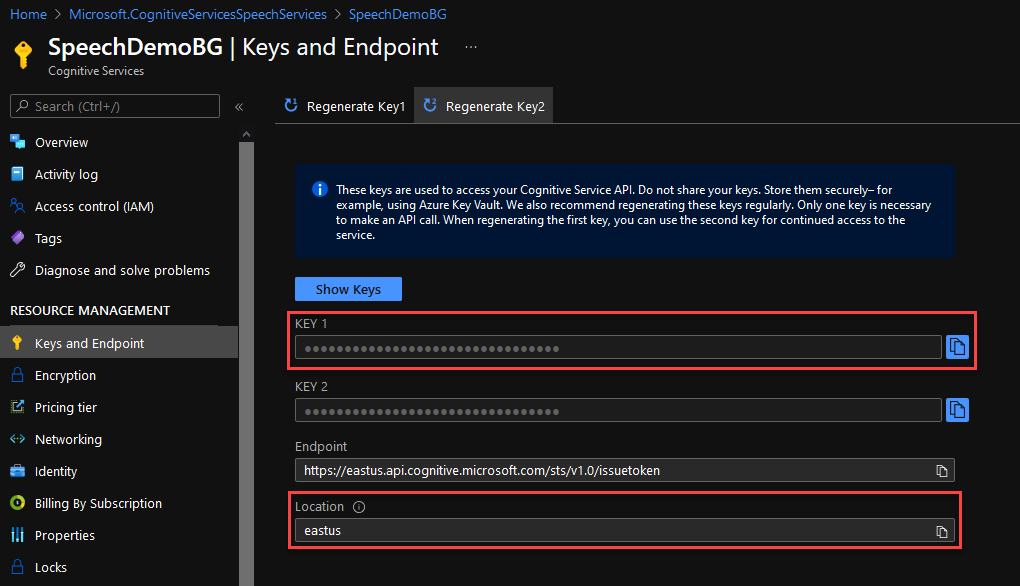
Implement
Open your existing project in Visual Studio. If you are not working on an existing project, simply create a C# (.NET Core) Console app.
Navigate to Project -> Manage NuGet Packages, find and install the Microsoft.CognitiveServices.Speech package.
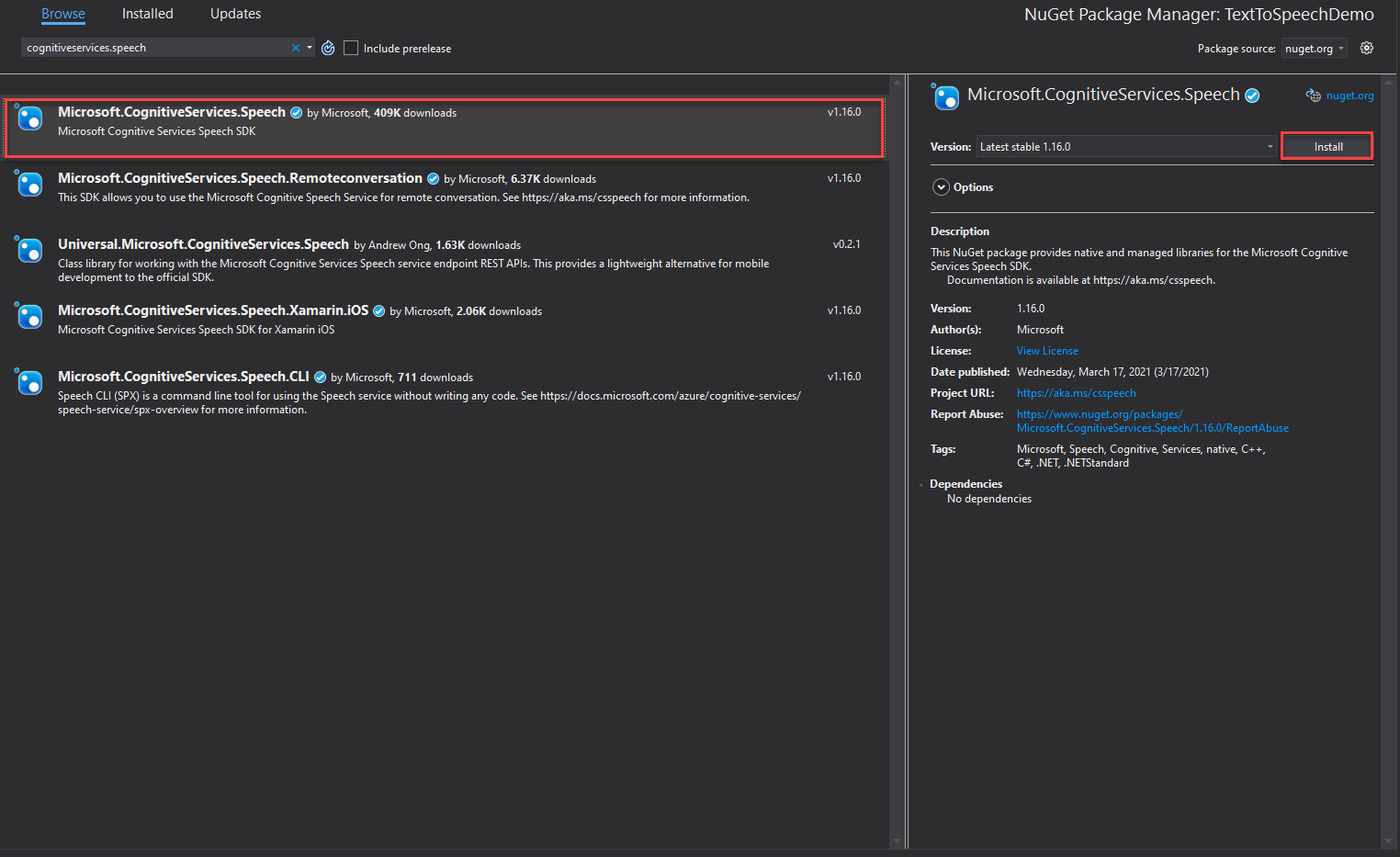
Open the class that you need to implement the speech synthesizer in. For a new project you can use the Program.cs.
Add the using statements you see below at the top of the file.
using System.Threading.Tasks; using Microsoft.CognitiveServices.Speech;
Replace your Main with the code below. Do not worry about the SynthesizeAudioAsync function, we will implement it in the next step. The input argument of the function is the text that is going to get synthesized, you can change this to anythin you like.
static async Task Main() { await SynthesizeAudioAsync("Sample text to get synthesized."); }
This is the function that connects to the Azure resource and synthesizes the text. Implement it under your Main function. The first argument of the FromSubscription is your key and the second your location.
static async Task SynthesizeAudioAsync(string textToSpeech) { var config = SpeechConfig.FromSubscription("7be282a06KEY_HEREb37d0c8f4a34", "eastus"); using var synthesizer = new SpeechSynthesizer(config); await synthesizer.SpeakTextAsync(textToSpeech); }
If you would like to try out more examples, or even output your spoken text to a file follow this link.
Test
Here is a sample of the output from the code above.
This is how you can rapidly intergrade a speech synthesizer to your project!