Get specific answers through the use of choice prompts
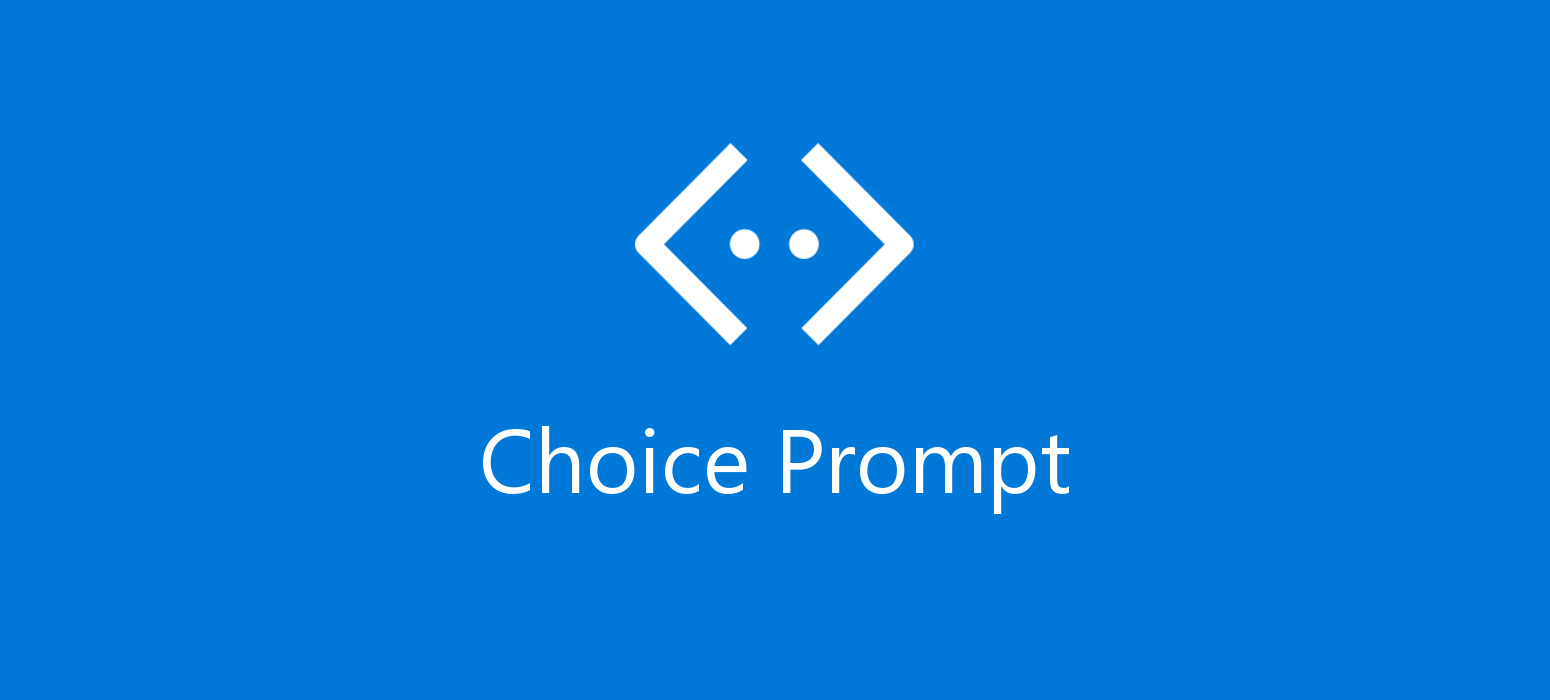
Choice Prompt gives you the ability to have full controll over the user’s answers. It provides the user with a list of options that he is allowed to chose from. It has an intergraded validator in case the user tries to say something that is not in the list of choices.
Why Choice Prompt?
Choice prompt gives a better sense of understanding to the user over the given question, because all the possible answers are already provided to him. It also gives complete control to the developer over the possible outcomes of that question. However, because of the nature of this prompt, you might need to avoid it, if your bot is published entirely in voice controlled channels, like a personal assistance. It works better if the user has a way to observe the answers.
The intergraded validator can be changed. As is, it checks if the user input matches any of the choices and if it does, it returns that as the answer. If not, the user gets re-prompted with the same question.
Create
In this example we have a weather bot. We are prompting the user for the day of the week that he would like the weather forecast for. You can follow through using the core bot sample. You can grab the sample from the GitHub repo, or directly from Azure using this post.
Add this using at the top of the file.
using Microsoft.Bot.Builder.Dialogs.Choices;
In the dialog section, add the ChoicePrompt dialog.
AddDialog(new ChoicePrompt(nameof(ChoicePrompt)));
Here is how you create the prompt. The string msgText contains the question of the prompt. The dayChoice list of type Choice contains all the answers. If the validator detects that user input does not satisfy any possible answer, the user is re-promped and msgText is replaced with retryText. There is also an optional Style option that can be used like this: “Style = ListStyle.HeroCard”. You can find all the possible styles here. Ignoring the style option, will leave its value at default, which is what we are doing below. It is important to know that the style shown through the emulator does not completely reflect the style in the published channels. Each cannel might present the data differently.
var msgText = "What days weather forecast would you like?"; var promptMessage = MessageFactory.Text(msgText, msgText, InputHints.ExpectingInput); var retryText = $"Please choose one option.\n\n{msgText}"; var retryPromptText = MessageFactory.Text(retryText, retryText, InputHints.ExpectingInput); var dayChoice = new List<Choice>() { new Choice("Monday"), new Choice("Tuesday"), new Choice("Wednesday"), new Choice("Thursday"), new Choice("Friday"), new Choice("Saturday"), new Choice("Sunday") }; return await stepContext.PromptAsync(nameof(ChoicePrompt), new PromptOptions { Prompt = promptMessage, Choices = dayChoice, RetryPrompt = retryPromptText }, cancellationToken);
In order to get the answer form the next prompt, you can use this line. You can not get the Results value directly, so you need to cast it to FoundChoice.
Day = ((FoundChoice)stepContext.Result).Value;
Test
After implementing the above step, your results should look like this. The user can either press on any of the answers, or write something in the input text box.
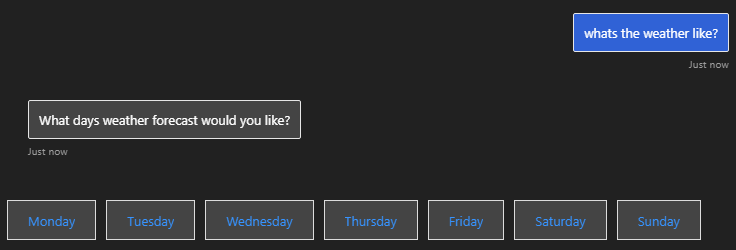
If the user enters an invalid answer, he is re-prompted with the retry message.
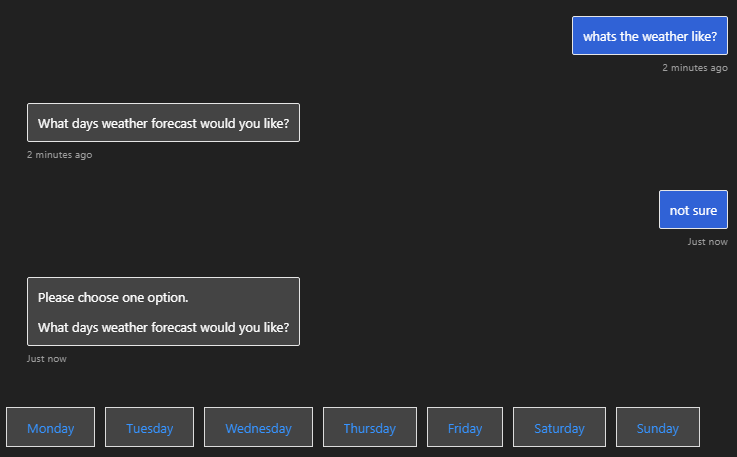
That is how you can implement Choice prompt to your bot, to improve your user’s experience.